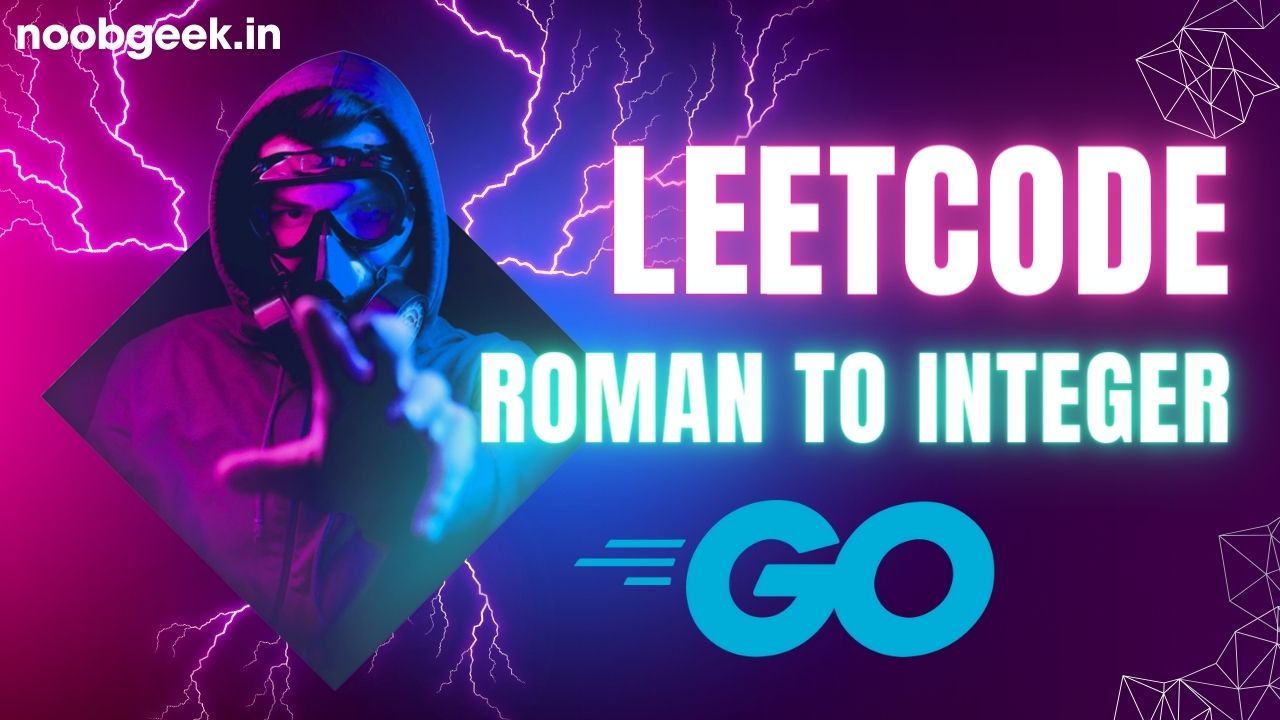
Roman to Integer | Leetcode | Golang Solution with Explaination
Roman numerals are represented by seven different symbols: I
, V
, X
, L
, C
, D
and M
.
Symbol Value I 1 V 5 X 10 L 50 C 100 D 500 M 1000
For example, 2
is written as II
in Roman numeral, just two ones added together. 12
is written as XII
, which is simply X + II
. The number 27
is written as XXVII
, which is XX + V + II
.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not IIII
. Instead, the number four is written as IV
. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as IX
. There are six instances where subtraction is used:
I
can be placed beforeV
(5) andX
(10) to make 4 and 9.X
can be placed beforeL
(50) andC
(100) to make 40 and 90.C
can be placed beforeD
(500) andM
(1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
Example 1:
Input: s = "III" Output: 3 Explanation: III = 3.
Example 2:
Input: s = "LVIII" Output: 58 Explanation: L = 50, V= 5, III = 3.
Example 3:
Input: s = "MCMXCIV" Output: 1994 Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
Constraints:
1 <= s.length <= 15
s
contains only the characters('I', 'V', 'X', 'L', 'C', 'D', 'M')
.- It is guaranteed that
s
is a valid roman numeral in the range[1, 3999]
.
Solution:
func romanToInt(s string) int { // Define a map to store the numeric values of each Roman symbol symbols_map := map[string]int{ "I": 1, "V": 5, "X": 10, "L": 50, "C": 100, "D": 500, "M": 1000, } // Initialize the result value v := 0 // Store the previous symbol to handle subtraction cases prev := "" // Loop through each character in the input string for _, c := range s { // Check if the current symbol's value is greater than the previous one if symbols_map[string(c)] > symbols_map[prev] { // If so, subtract the previous symbol's value from the result v = v - symbols_map[prev] // Add the difference between the current symbol's value and the previous one to the result v = v + (symbols_map[string(c)] - symbols_map[prev]) } else { // If not, simply add the current symbol's value to the result v += symbols_map[string(c)] } // Update the previous symbol for the next iteration prev = string(c) } // Return the final integer value return v }
Explaination:
- Symbol Mapping: The function starts by defining a mapping between Roman symbols and their corresponding integer values using a Go map.
- Initialization: It initializes the result variable v to 0 and a variable prev to store the previous symbol encountered during iteration.
- Iteration: The function iterates through each character in the input string s.
- Comparing Symbols: For each symbol, it compares its numeric value with the previous one. If the current symbol's value is greater than that of the previous symbol, it implies a subtraction case (e.g., IV = 5 - 1 = 4).
- Updating Result: Accordingly, it updates the result variable v by subtracting the value of the previous symbol and then adding the difference between the current symbol's value and the previous one. If no subtraction is needed, it simply adds the current symbol's value to the result.
- Updating Previous Symbol: Finally, it updates the prev variable to store the current symbol for the next iteration.
- Result: Once all symbols are processed, it returns the final integer value representing the input Roman numeral string.
Thanks for reading this ❤.
Post a comment
Get your FREE PDF on "100 Ways to Try ChatGPT Today"
Generating link, please wait for: 60 seconds
Comments
Gonzalez Ingrid
Monday, March 3, 2025 at 7:33 AM ISTLosing a significant amount of money, especially after years of hard work, was something I never expected to experience. As a pilot, I had spent countless hours away from home, saving diligently from my earnings. When I decided to invest my 133K CAD gratuity with ForexTilt, I thought it was a smart decision that would help grow my savings. The platform seemed promising, and I was confident in my choice. However, everything quickly began to fall apart. The platform’s performance started to decline, and before I knew it, my entire 133K CAD investment had vanished. I was in shock.This money wasn’t just an investment; it was a product of years of sacrifice and hard work. Losing it was heartbreaking, and I was left feeling utterly helpless. At first, I tried to convince myself that it was a temporary issue, but the reality soon set in: I had been scammed. The disappointment and frustration were overwhelming, and I had no idea where to turn for help.I felt an overwhelming sense of loss and w